Set up development environment
Introduction
This tutorial will help you set up a local environment for developing blueprints and testing nano contracts. You should have already completed unit tests for your blueprints; this environment will now allow you to conduct integration tests.
Overview of the task
The following diagram illustrates the architecture of the environment we will set up:
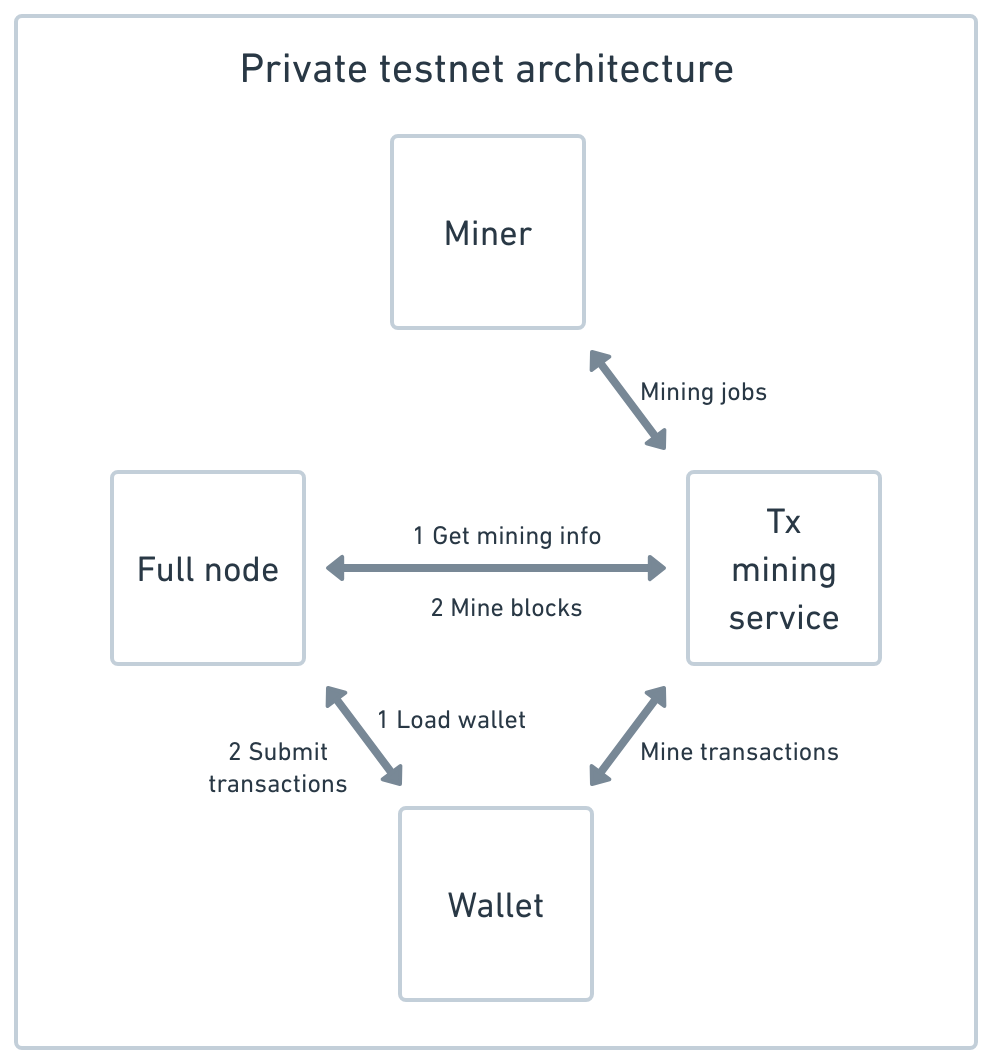
After setting up the development environment, we will perform integration tests for a blueprint, specifically using the SwapDemo blueprint. This blueprint is already included in the Hathor core source code, but it is not yet registered for use.
Sequence of steps
- Set up Hathor core (full node).
- Set up wallet application.
- Register nano contract.
- Set up transaction mining service.
- Set up miner.
- Test nano contract.
- Connect to public testnet.
- Submit blueprint to public testnet.
Task execution
Now, it's time to get your hands dirty. In this section, we will describe the steps in detail.
Step 1: install Hathor core
For developing and testing blueprints, it's easier to have access to the local file system so you can quickly modify the blueprint file and iterate. You must install Hathor core (full node) from source code. See: Install full node from source code and then install the correct branch:
git clone -b experimental/nano-testnet-v1.6.1 https://github.com/HathorNetwork/hathor-core.git
Before running the full node, you should add the following setting on hathor/conf/nano_testnet.yml
:
REWARD_SPEND_MIN_BLOCKS: 3
REWARD_SPEND_MIN_BLOCKS
sets how many new blocks are needed for a block reward to become available (unlocked). The default (300) is too long for local development as we want to have HTR available for our deployment.
You can run the full node with the following command:
./hathor-cli run_node --wallet-index --nc-history-index --allow-mining-without-peers --status 8080 --memory-storage --x-localhost-only --nano-testnet
This will expose the API on port 8080. Also, it will use memory storage, so all transactions and blocks will be lost in case you restart the node. For persistent storage, you can remove --memory-storage
and use --data ./my_data_folder
.
Step 2: set up wallet application
Now that your full node is running, let's take care of the wallet application. In this tutorial, we will use Hathor desktop wallet. Start your Hathor desktop wallet.
On the settings tab, change the server and connect to http://locahost:8080/v1a/
. It will ask you to confirm switching to a testnet. Once it's done and the wallet loads, you can write down your wallet address (it should start with H
), which will be needed for the next steps.
Step 3: register nano contract
At this moment, the easiest way to test nano contracts is to have them registered beforehand so it can be called on the wallet. There is a way to create nano contracts dynamically, but for local tests it's easier to pre-register the contract.
Your code should be on the hathor/nanocontracts/blueprints/
directory. You can check that the swap_demo.py
is already there. Each blueprint needs a unique id, which is a 32-byte (64 hex chars) identifier, similar to transaction ids. This can be any random number id. You can generate one using this website by typing any text on the input.
First, export your contract on the hathor/nanocontracts/blueprints/__init__.py
file. Make sure to replace the file and class names with the appropriate ones.
--- a/hathor/nanocontracts/blueprints/__init__.py
+++ b/hathor/nanocontracts/blueprints/__init__.py
@@ -16,6 +16,7 @@ from typing import TYPE_CHECKING, Type
from hathor.nanocontracts.blueprints.bet import Bet
from hathor.nanocontracts.blueprints.dozer_pool import Dozer_Pool
+from hathor.nanocontracts.blueprints.swap_demo import SwapDemo
if TYPE_CHECKING:
from hathor.nanocontracts.blueprint import Blueprint
@@ -24,9 +25,11 @@ if TYPE_CHECKING:
_blueprints_mapper: dict[str, Type["Blueprint"]] = {
"Bet": Bet,
"Dozer_Pool": Dozer_Pool,
+ "SwapDemo": SwapDemo,
}
__all__ = [
"Bet",
"Dozer_Pool",
+ "SwapDemo",
]
Then, on hathor/conf/nano_testnet.yml
, register the blueprint. This will be the blueprint id.
--- a/hathor/conf/nano_testnet.yml
+++ b/hathor/conf/nano_testnet.yml
@@ -4,6 +4,8 @@ NETWORK_NAME: nano-testnet-alpha
BOOTSTRAP_DNS:
- alpha.nano-testnet.hathor.network
+REWARD_SPEND_MIN_BLOCKS: 3
+
# Genesis stuff
GENESIS_OUTPUT_SCRIPT: 76a91478e804bf8aa68332c6c1ada274ac598178b972bf88ac
GENESIS_BLOCK_TIMESTAMP: 1677601898
@@ -22,3 +24,4 @@ ENABLE_NANO_CONTRACTS: true
BLUEPRINTS:
3cb032600bdf7db784800e4ea911b10676fa2f67591f82bb62628c234e771595: Bet
27db2b0b1a943c2714fb19d190ce87dc0094bba463b26452dd98de21a42e96a0: Dozer_Pool
+ 41c4c4f09455f89a00345c8ea50c166d7e41cf80952e76db41f5d7a3044298e7: SwapDemo
41c4c4f09455f89a00345c8ea50c166d7e41cf80952e76db41f5d7a3044298e7
is the SwapDemo blueprint id.
Step 4: Set up transaction mining service
This service mines transactions and, when idle, mines blocks as well. We can use the docker images available, just remember to update with your own testnet address:
docker run -it -p 9000:9000 -p 9080:9080 hathornetwork/tx-mining-service http://host.docker.internal:8080 --address WiN5T6JoUiJgix7KLrKTefDhEJb4JPPPgV --testnet --stratum-port 9000 --api-port 9080
If you are running on newer Macs, you may get the error docker: no matching manifest for linux/arm64/v8 in the manifest list entries
. To fix it, add this to the docker command: --platform linux/x86_64
.
This command uses host.docker.internal
to communicate with the localhost. This is a bit different on Linux: https://stackoverflow.com/a/43541732/2340855
The service will start and listen in two ports:
- 9000: Stratum API port, used by miners to get and submit mining jobs;
- 9080: HTTP API port, used to get status and submit transactions.
To check it's working, use:
curl http://localhost:9080/mining-status`.
Step 5: set up miner
Finally, you can connect a miner to the transaction mining service. Also remember to update the address:
docker run -ti hathornetwork/cpuminer -t 1 -a sha256d -o stratum+tcp://host.docker.internal:9000 --coinbase-addr WiN5T6JoUiJgix7KLrKTefDhEJb4JPPPgV`
You should start see some blocks being mined and some HTR balance on your wallet.
Step 6: test nano contract
Now that everything is up and running, we can start testing. On your wallet, go to settings and click "Change mining service". Change it to http://localhost:9080
. This will make sure you are using your local transaction mining service.
When testing, you can make modifications on the nano contracts file and restart the full node. If you are using memory storage, you will also have to restart the transaction mining service. The miner should reconnect automatically.
We will use the desktop wallet to make the final test on the SwapDemo contract. Here's the video:
Step 7: connect to public testnet
To migrate from your local development environment to the public test environment, use the following URLs:
Public full node in nano-testnet:
https://node1.nano-testnet.hathor.network/v1a/
Tx mining service in nano-testnet:
https://txmining.nano-testnet.hathor.network/
Explorer in nano-testnet:
https://explorer.alpha.nano-testnet.hathor.network/
Command to run a local full node on nano-testnet:
./hathor-cli run_node --wallet-index --status 8080 --nano-testnet --nc-history-index --data ./data/
Step 8: submit blueprint to public testnet
In this initial stage of the Nano Contracts public testnet, sending new blueprints to the network is restricted to Hathor Labs. The core team is testing the best way to allow anyone to send their blueprint to the network in the near future.
If you want to have your blueprint added, you can open a pull request to the hathor-core
repository (https://github.com/HathorNetwork/hathor-core). The blueprint code should be in folder hathor/nanocontracts/blueprints/
. Use the branch experimental/nano-testnet-v1.6
and open the pull request to it.
Since only the Hathor core team can push to the hathor-core
repo, you need to fork the repository and open a pull request from it. This guide shows you how to do it.
Before submitting, we expect that you have tested your blueprint, not only with unit tests but also using a local development environment for end-to-end testing.
If you have any questions, you can contact the team on the #development
channel on Hathor Discord server].